Java Reference
In-Depth Information
The remaining types of loops are
while
and
do
. As with
for
loops,
while
and
do
loops
enable a block of Java code to be executed repeatedly until a specific condition is met.
Whether you use a
for
,
while
, or
do
loop is mostly a matter of your programming style.
while
Loops
The
while
loop repeats a statement for as long as a particular condition remains
true
.
Here's an example:
while (i < 13) {
x = x * i++; // the body of the loop
}
The condition that accompanies the
while
keyword is a Boolean expression—
i < 13
in
the preceding example. If the expression returns
true
, the
while
loop executes the body
of the loop and then tests the condition again. This process repeats until the condition is
false
.
Although the preceding loop uses opening and closing braces to form a block statement,
the braces are not needed because the loop contains only one statement:
x = x * i++
.
Using the braces does not create any problems, though, and the braces will be required if
you add another statement inside the loop later.
4
The
ArrayCopier
application in Listing 4.4 uses a
while
loop to copy the elements of an
array of integers (in
array1
) to an array of float variables (in
array2
), casting each ele-
ment to a
float
as it goes. The one catch is that if any of the elements in the first array
is 1, the loop immediately exits at that point.
LISTING 4.4
The Full Text of
ArrayCopier.java
1: class ArrayCopier {
2: public static void main(String[] arguments) {
3: int[] array1 = { 7, 4, 8, 1, 4, 1, 4 };
4: float[] array2 = new float[array1.length];
5:
6: System.out.print(“array1: [ “);
7: for (int i = 0; i < array1.length; i++) {
8: System.out.print(array1[i] + “ “);
9: }
10: System.out.println(“]”);
11:
12: System.out.print(“array2: [ “);
13: int count = 0;
14: while ( count < array1.length && array1[count] != 1) {
15: array2[count] = (float) array1[count];

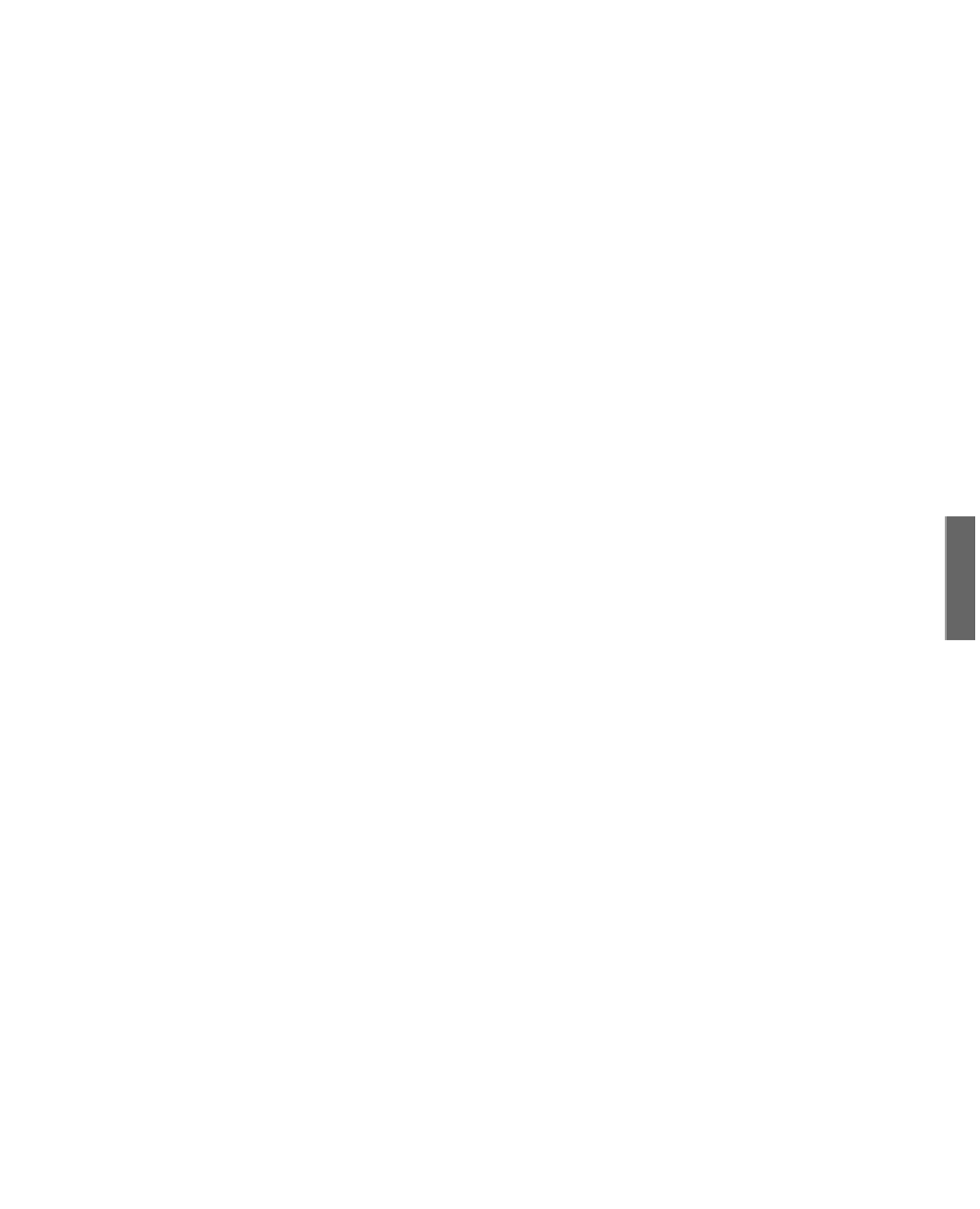