Java Reference
In-Depth Information
In Listing 4.2, the
DayCounter
application takes two arguments, a month and a year, and
displays the number of days in that month. A
switch
statement,
if
statements, and
else
statements are used.
LISTING 4.2
The Full Text of
DayCounter.java
1: class DayCounter {
2: public static void main(String[] arguments) {
3: int yearIn = 2008;
4: int monthIn = 1;
5: if (arguments.length > 0)
6: monthIn = Integer.parseInt(arguments[0]);
7: if (arguments.length > 1)
8: yearIn = Integer.parseInt(arguments[1]);
9: System.out.println(monthIn + “/” + yearIn + “ has “
10: + countDays(monthIn, yearIn) + “ days.”);
11: }
12:
13: static int countDays(int month, int year) {
14: int count = -1;
15: switch (month) {
16: case 1:
17: case 3:
18: case 5:
19: case 7:
20: case 8:
21: case 10:
22: case 12:
23: count = 31;
24: break;
25: case 4:
26: case 6:
27: case 9:
28: case 11:
29: count = 30;
30: break;
31: case 2:
32: if (year % 4 == 0)
33: count = 29;
34: else
35: count = 28;
36: if ((year % 100 == 0) & (year % 400 != 0))
37: count = 28;
38: }
39: return count;
40: }
41: }
4

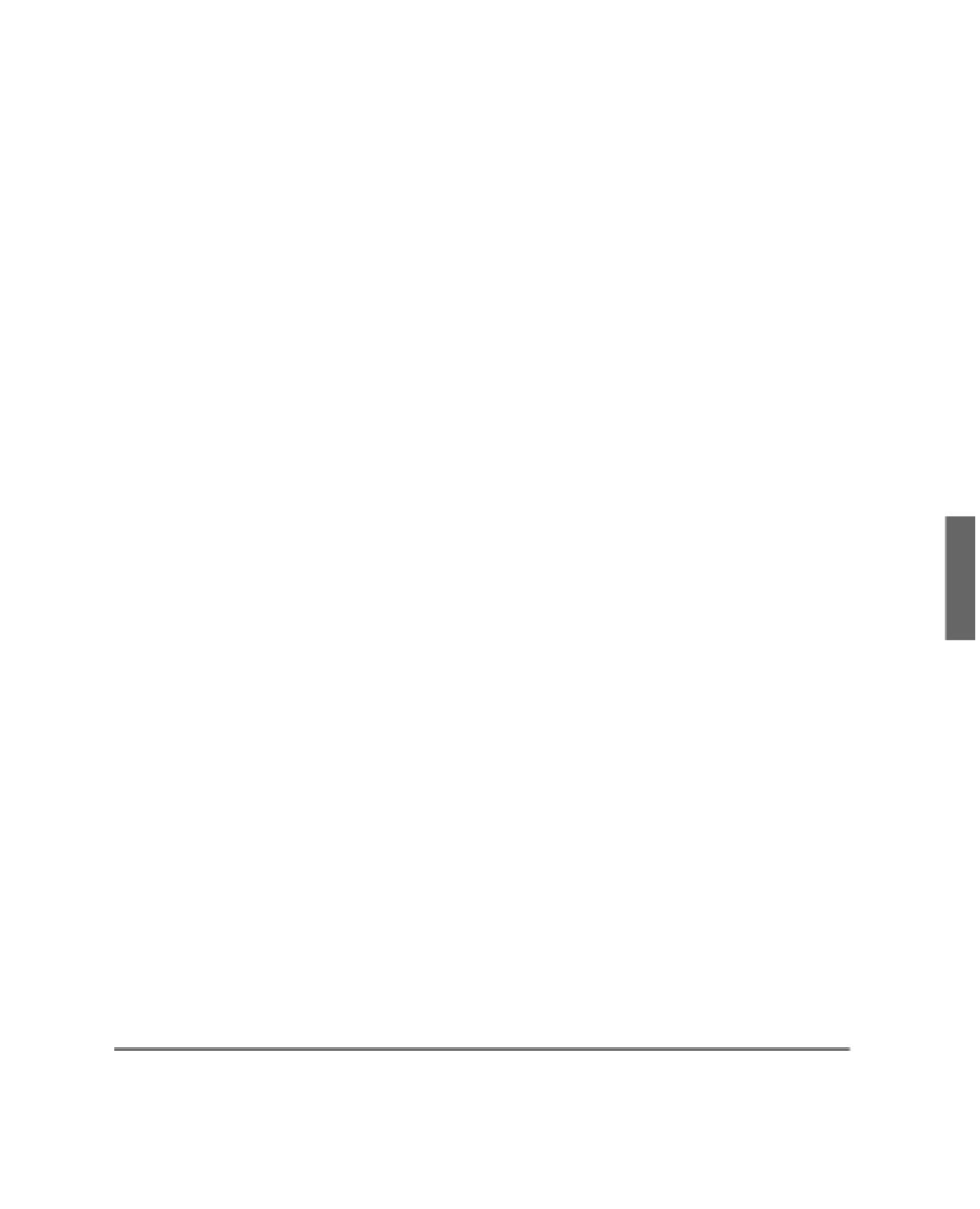