Java Reference
In-Depth Information
series[1] = new Integer(3);
series[2] = new Integer(5);
You can create and initialize an array at the same time by enclosing the elements of the
array inside braces, separated by commas:
Point[] markup = { new Point(1,5), new Point(3,3), new Point(2,3) };
Each of the elements inside the braces must be the same type as the variable that holds
the array. When you create an array with initial values in this manner, the array will be
the same size as the number of elements you include within the braces. The preceding
example creates an array of
Point
objects named
markup
that contains three elements.
Because
String
objects can be created and initialized without the
new
operator, you can
do the same when creating an array of strings:
String[] titles = { “Mr.”, “Mrs.”, “Ms.”, “Miss”, “Dr.” };
The preceding statement creates a five-element array of
String
objects named
titles
.
All arrays have an instance variable named
length
that contains the number of elements
in the array. Extending the preceding example, the variable
titles.length
contains the
value
4
.
Accessing Array Elements
After you have an array with initial values, you can retrieve, change, and test the values
in each slot of that array. The value in a slot is accessed with the array name followed by
a subscript enclosed within square brackets. This name and subscript can be put into
expressions, as in the following:
testScore[40] = 920;
The first element of an array has a subscript of
0
rather than
1
, so an array with 12 ele-
ments has array slots accessed by using subscripts
0
through
11
.
The preceding statement sets the 41st element of the
testScore
array to a value of
920
.
The
testScore
part of this expression is a variable holding an array object, although it
also can be an expression that results in an array. The subscript expression specifies the
slot to access within the array.
All array subscripts are checked to make sure that they are inside the array's boundaries
as specified when the array was created. In Java, it is impossible to access or assign a
value to an array slot outside the array's boundaries, avoiding problems that result from
overrunning the bounds of an array in C-type languages. Note the following two state-
ments:
float[] rating = new float[20];
rating[20] = 3.22F;
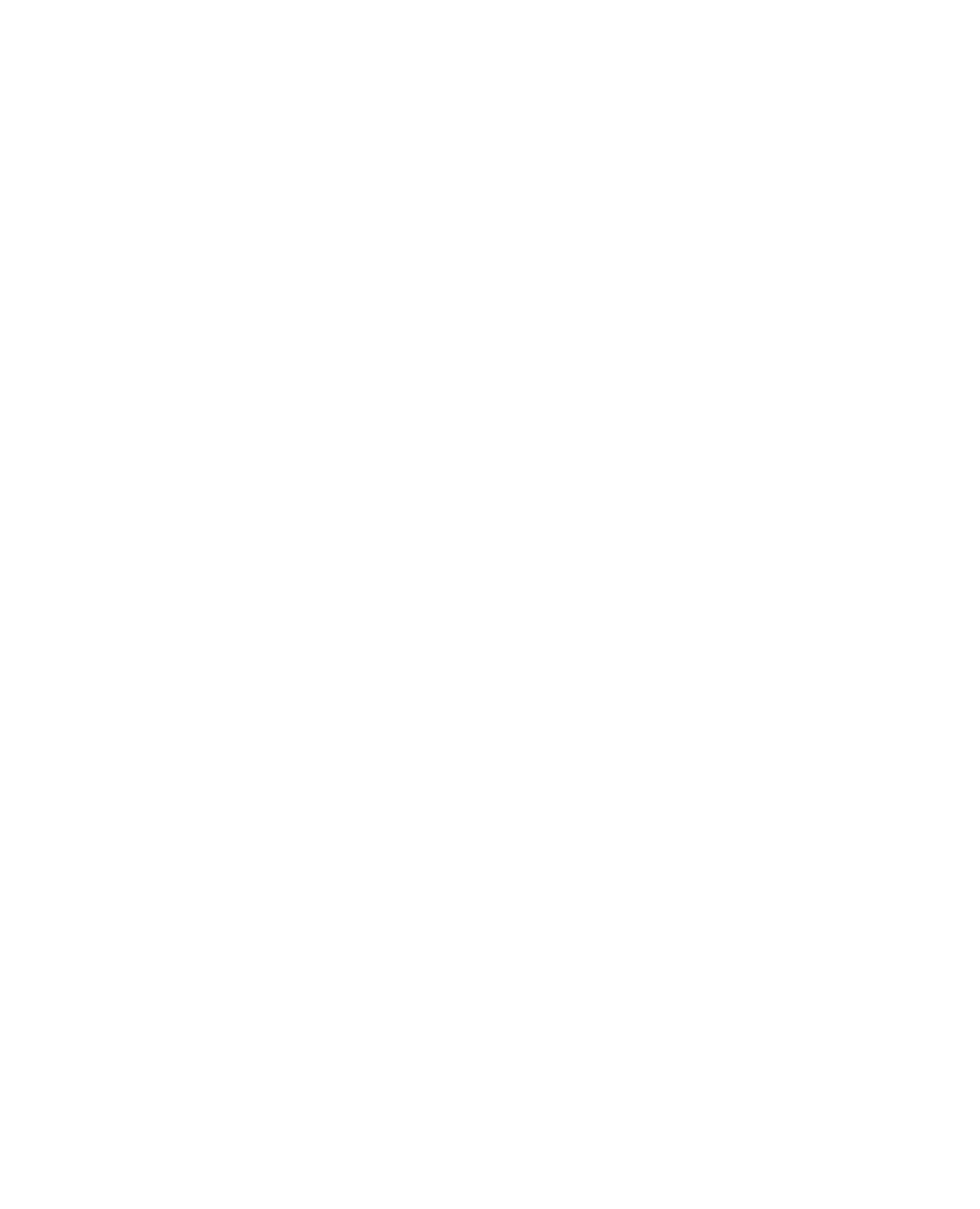