Databases Reference
In-Depth Information
// Prepare the UPDATE statement for multiple executions
sql = "UPDATE employees SET salary = salary * 1.05" +
"WHERE id = ?";
prepStmt = con.prepareStatement(sql);
// Execute the UPDATE statement for each
// value of index between 0 and 9
for (int index = 0; index < 10; index++) {
prepStmt.setInt(1, index);
prepStmt.executeUpdate();
}
// Manual commit
con.commit();
// Execute a SELECT statement. A prepare is unnecessary
// because it's only executed once.
sql = "SELECT id, name, salary FROM employees";
// Fetch the data
resultSet = stmt.executeQuery(sql);
while (resultSet.next()) {
System.out.println("Id: " + resultSet.getInt(1) +
"Name: " + resultSet.getString(2) +
"Salary: " + resultSet.getInt(3));
}
System.out.println();
// Close the result set
resultSet.close();
}
finally {
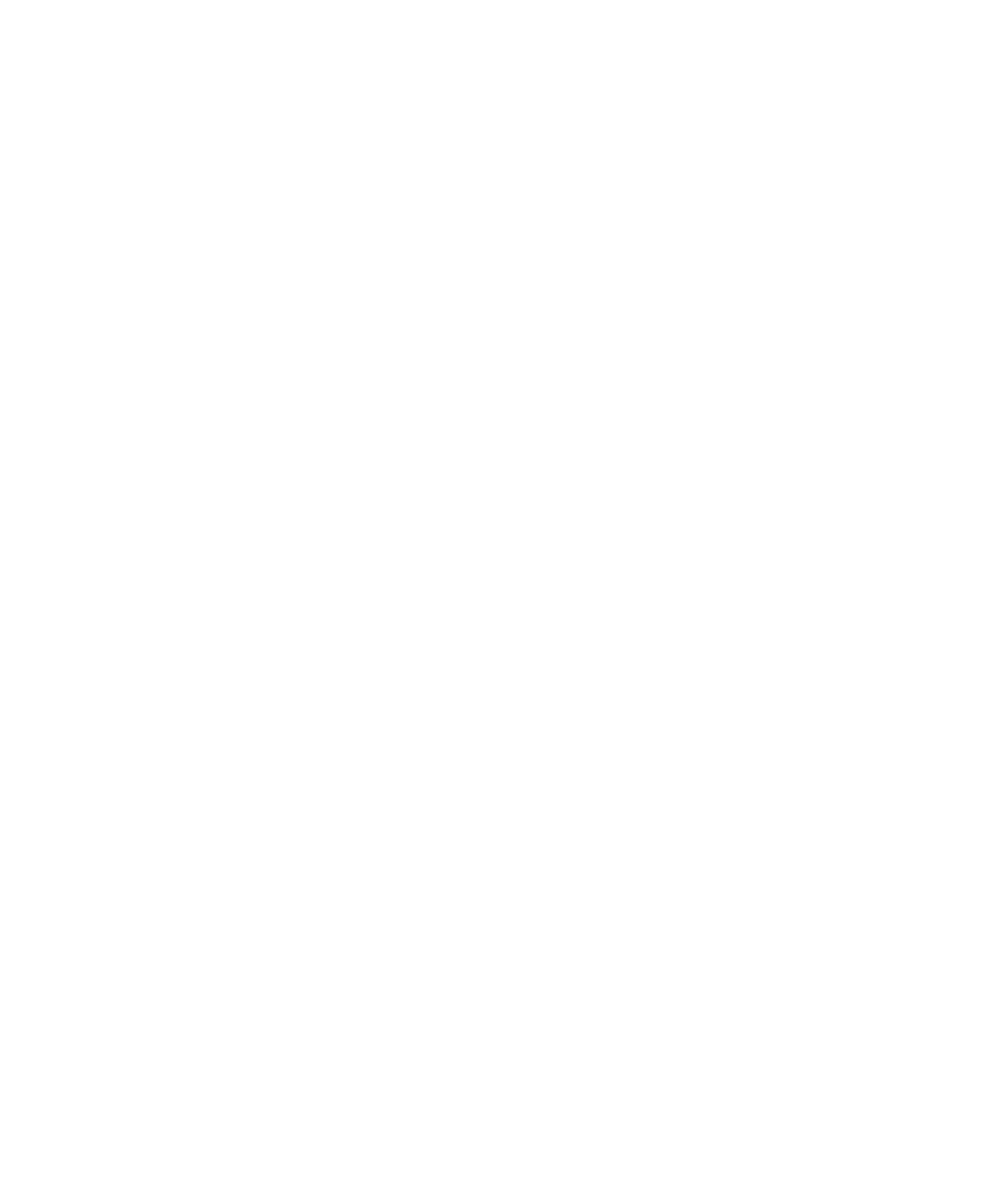