Java Reference
In-Depth Information
public class LicenseManager {
Map<LicenseType, String> licenseMap =
new HashMap<LicenseType, String>();
public LicenseManager() {
LicenseType type = new LicenseType();
type.setType("demo-license-key");
licenseMap.put(type, "ABC-DEF-PQR-XYZ");
}
public Object getLicenseKey(LicenseType licenseType) {
return licenseMap.get(licenseType);
}
public void setLicenseKey(LicenseType licenseType,
String licenseKey) {
licenseMap.put(licenseType, licenseKey);
}
}
class LicenseType {
private String type;
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
@Override
public int hashCode() {
int res = 17;
res = res * 31 + type == null ? 0 : type.hashCode();
return res;
}
@Override
public boolean equals(Object arg) {
if (arg == null || !(arg instanceof LicenseType)) {
return false;
}
if (type.equals(((LicenseType) arg).getType())) {
return true;
}
return false;
}
}
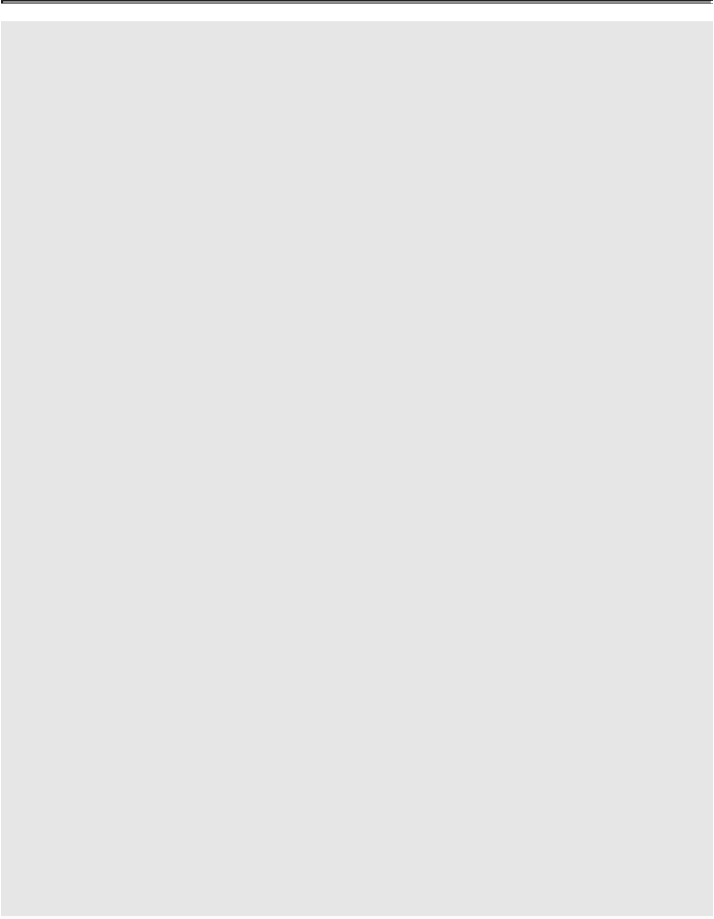
