Java Reference
In-Depth Information
The
storeDateInDB()
method accepts an untrusted date argument and attempts to
make a defensive copy using its
clone()
method. This allows an attacker to take control
of the program by creating a malicious date class that extends
Date
. If the attacker's code
runs with the same privileges as
store-DateInDB()
, the attacker merely embeds mali-
cious code inside their
clone()
method:
class MaliciousDate extends java.util.Date {
@Override
public MaliciousDate clone() {
// malicious code goes here
}
}
If,however,theattackercanonlyprovideamaliciousdatewithlessenedprivileges,the
attacker can bypass validation but still confound the remainder of the program. Consider
this example:
public class MaliciousDate extends java.util.Date {
private static int count = 0;
@Override
public long getTime() {
java.util.Date d = new java.util.Date();
return (count++ == 1) ? d.getTime() : d.getTime() - 1000;
}
}
Thismaliciousdatewillappeartobeabenigndateobjectthefirsttimethat
getTime()
isinvoked.Thisallowsittobypassvalidationinthe
storeDateInDB()
method.However,
the time that is actually stored in the database will be incorrect.
Compliant Solution
This compliant solution avoids using the
clone()
method. Instead, it creates a new
java.util.Date
object that is subsequently used for access control checks and insertion
into the database.



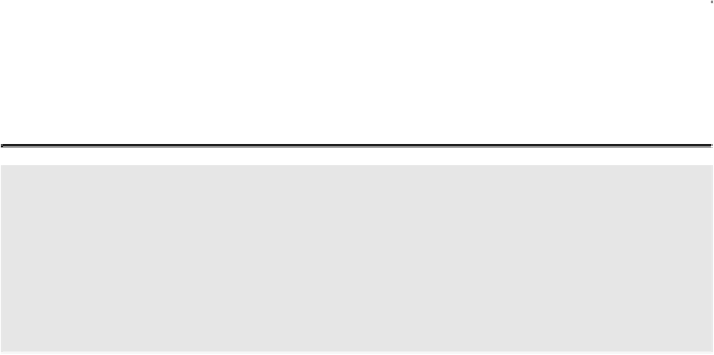


