Java Reference
In-Depth Information
Compliant Solution
This compliant solution defines a
ValidateOutput
class that normalizes the output to
a known character set, performs output sanitization using a whitelist, and encodes any
unspecified data values to enforce a double-checking mechanism. Note that the required
whitelisting patterns will vary according to the specific needs of different fields [OWASP
2013].
public class ValidateOutput {
// Allows only alphanumeric characters and spaces
private static final Pattern pattern =
Pattern.compile("^[a-zA-Z0-9\\s]{0,20}$");
// Validates and encodes the input field based on a whitelist
public String validate(String name, String input)
throws ValidationException {
String canonical = normalize(input);
if (!pattern.matcher(canonical).matches()) {
throw new ValidationException("Improper format in " +
name + " field");
}
// Performs output encoding for nonvalid characters
canonical = HTMLEntityEncode(canonical);
return canonical;
}
// Normalizes to known instances
private String normalize(String input) {
String canonical =
java.text.Normalizer.normalize(input,
Normalizer.Form.NFKC);
return canonical;
}
// Encodes nonvalid data
private static String HTMLEntityEncode(String input) {
StringBuffer sb = new StringBuffer();
for (int i = 0; i < input.length(); i++) {
char ch = input.charAt(i);
if (Character.isLetterOrDigit(ch) ||
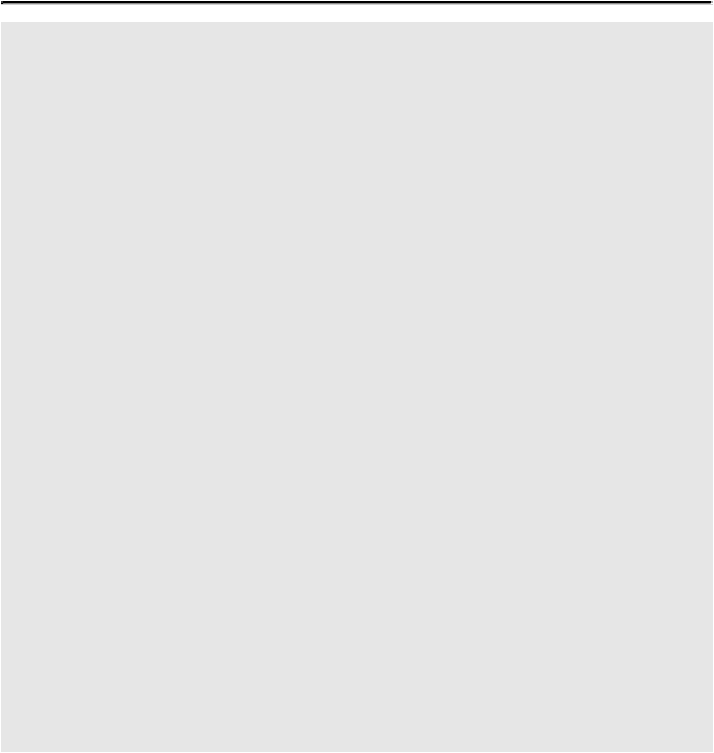
