Java Reference
In-Depth Information
public static void main(String[] args) {
// Single ArrayList
System.out.println(display(new ArrayList<Integer>()));
// Array of lists
List<?>[] invokeAll = new List<?>[] {
new ArrayList<Integer>(),
new LinkedList<String>(),
new Vector<Integer>()};
for (List<?> list : invokeAll) {
System.out.println(display(list));
}
}
}
At compile time, the type of the object array is
List
. The expected output is
Ar-
rayList
,
ArrayList
,
LinkedList
, and
List is not recognized
(because
java.util.Vector
is neither an
ArrayList
nor a
LinkedList
). The actual output is
Ar-
rayList
followed by
List is not recognized
repeated three times. The cause of
this unexpected behavior is that overloaded method invocations are affected
only
by the
compile-time type of their arguments:
ArrayList
for the first invocation and
List
for the
others.
Compliant Solution
This compliant solution uses a single
display
method and
instanceof
to distinguish
between different types. As expected, the output is
ArrayList
,
ArrayList
,
LinkedList
,
List is not recognized
:
public class Overloader {
private static String display(List<?> list) {
return (
list instanceof ArrayList ? "Arraylist" :
(list instanceof LinkedList ? "LinkedList" :
"List is not recognized")
);
}
public static void main(String[] args) {
// Single ArrayList
System.out.println(display(new ArrayList<Integer>()));
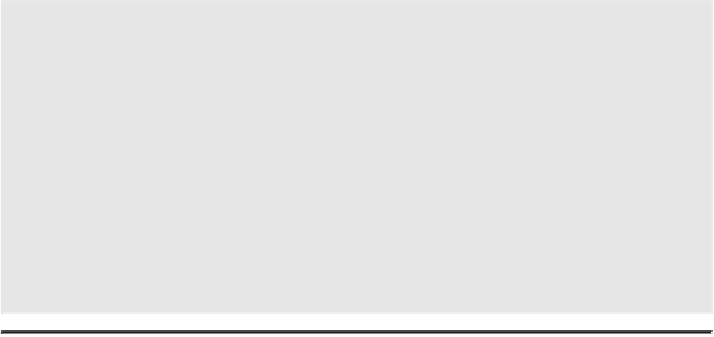
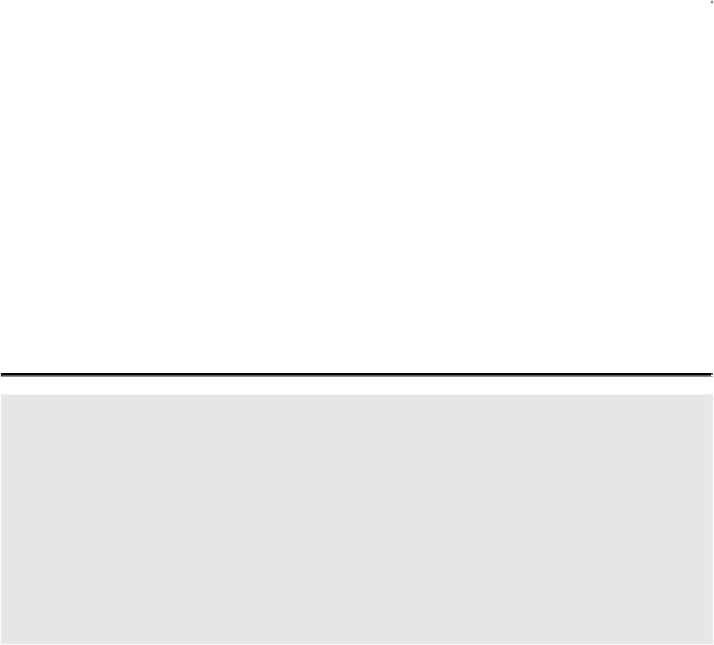
