Java Reference
In-Depth Information
public String processSingleString(String string) {
// ...
return string;
}
public String processStrings(String[] strings) {
String result = "";
int i = 0;
try {
while (true) {
result = result.concat(processSingleString(strings[i]));
i++;
}
} catch (ArrayIndexOutOfBoundsException e) {
// Ignore, we're done
}
return result;
}
This code uses an
ArrayIndexOutOfBoundsException
to detect the end of the array.
Unfortunately, because
ArrayIndexOutOfBoundsException
is a
RuntimeException
, it
could be thrown by
processSingleString()
without being declared in a
throws
clause.
So it is possible for
processStrings()
to terminate prematurely before processing all of
the strings.
Compliant Solution
This compliant solution uses a standard
for
loop to concatenate the strings.
public String processStrings(String[] strings) {
String result = "";
for (int i = 0; i < strings.length; i++) {
result = result.concat(processSingleString(strings[i]));
}
return result;
}



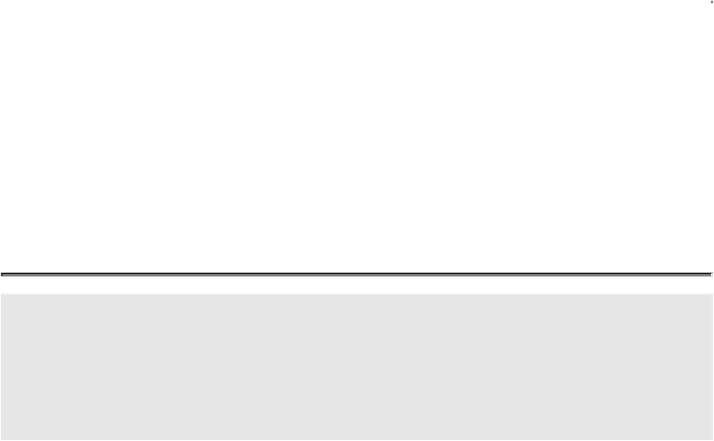


