Java Reference
In-Depth Information
As shown in Figure 11-8, the GUI contains 16 buttons, a text field, and a window. The
buttons and the text field are placed in the content pane of the window. The user enters
the input using the various buttons, and the program displays the result in the text field.
To create the 16 buttons, you use 16 reference variables of type
JButton
, and to create
thetextfield,youuseareferencevariableoftype
JTextField
. You also need a
reference variable to access the content pane of the window. As we did in previous
GUI programs (in Chapters 6, 8, and 10), we create the class containing the application
program by extending the definition of the
class
JFrame
, which also allows you to
create the necessary window to create the GUI. Thus, we use the following variables to
create the GUI components and to access the content pane of the window:
PROBLEM
ANALYSIS
AND GUI AND
ALGORITHM
DESIGN
private
JTextField displayText =
new
JTextField(30);
private
JButton[] button =
new
JButton[16];
Container pane = getContentPane(); //
to access the content pane
As you can see from Figure 11-8, the GUI components are nicely organized. To place
the GUI components as shown in the figure, we first set the layout of the content pane
to
null
andthenusethemethods
setSize
and
setLocation
to place the GUI
components at various locations in the content pane. The following statement instanti-
ates the
JTextField
object
displayText
and places it in the content pane:
displayText.setSize(200, 30);
displayText.setLocation(10, 10);
pane.add(displayText);
The size of
displayText
is set to
200
pixels wide and
30
pixels high and it is placed
at position
(10, 10)
in the content pane.
To assign labels to the buttons, rather than write 16 statements, we use an array of
strings and a loop. Consider the following statement:
1
1
private
String[] keys = {"7", "8", "9", "/",
"4", "5", "6", "*",
"1", "2", "3", "-",
"0", "C", "=", "+"};
Because the size of the
displayText
is
200
pixels wide and each row has four
JButtons
, we set the width of each
JButton
to
50
pixels wide. To keep the height
of each
JButton
the same as the height of
displayText
, we set the height of each
JButton
to
30
pixels.
The user enters input via the buttons. Therefore, each button can generate an action
event. To respond to the events generated by a button, we will create and register an
appropriate object. In Chapters 6, 8, and 10, we used the mechanism of the inner class
to create and register a listener object. In this program, we make the class containing
the application program implement the
interface
ActionListener
. Therefore, we
only need to provide the definition of the method
actionPerformed
, which will be
described later in this section. Because the class containing the application program
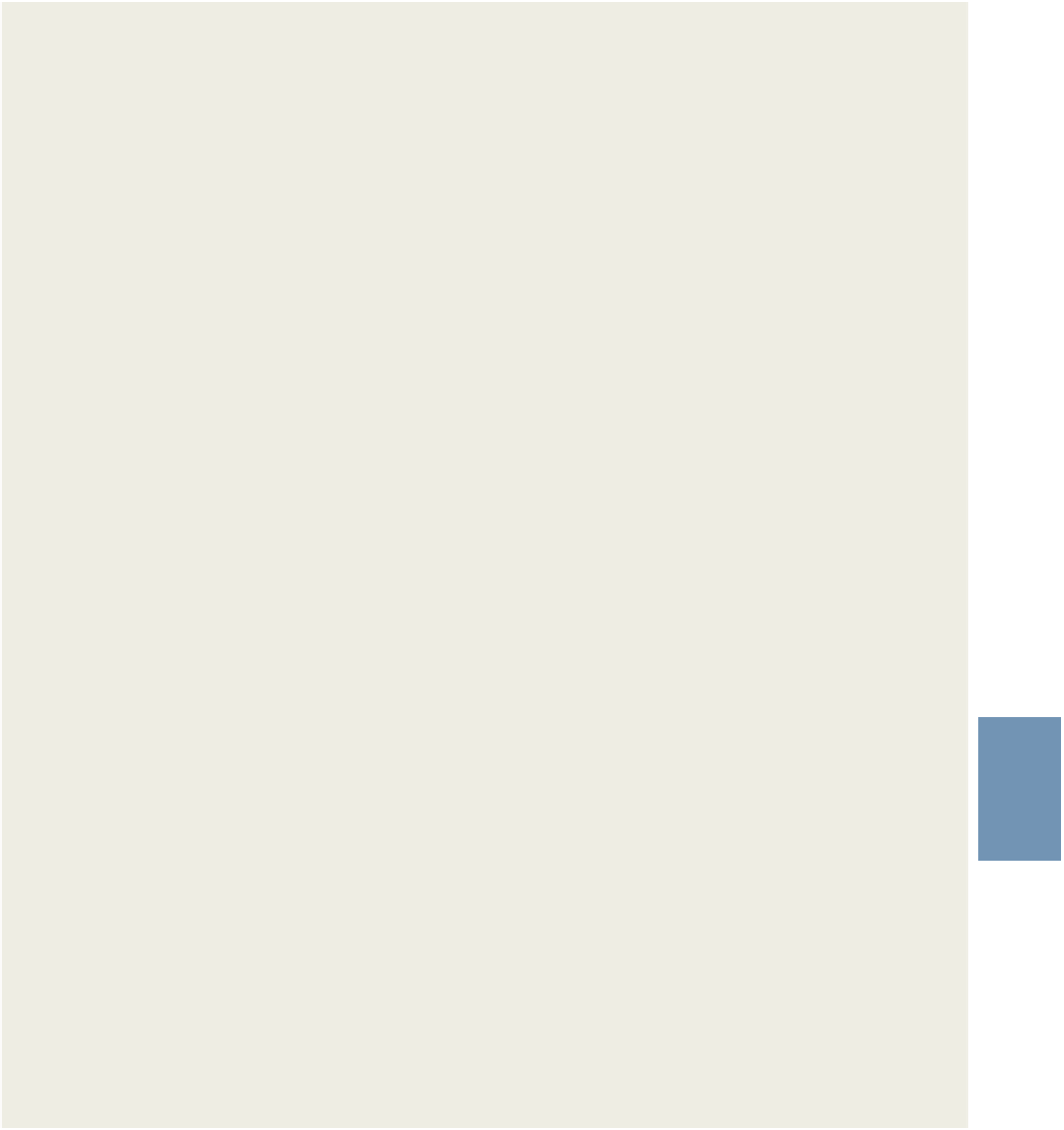
Search WWH ::

Custom Search