Java Reference
In-Depth Information
Chapter 6 discussed in detail how to handle action events. Recall that to handle an action
event, we do the following:
1. Create a class that implements the
interface
ActionListener
. For
example, in Chapter 6, for the
JButton calculateB
, we created the
class
CalculateButtonHandler
.
2. Provide the definition of the method
actionPerformed
within the class
that you created in Step 1. The method
actionPerformed
contains the
code that the program executes when the specific event is generated. For
example, in Chapter 6, when you click the
JButton calculateB
,the
program should calculate and display the area and perimeter of the rectangle.
3. Create and instantiate an object of the class created in Step 1. For
example, in Chapter 6, for the
JButton calculateB
, we created the
object
cbHandler
.
4. Register the event handler created in Step 3 with the object that generates
an action event using the method
addActionListener
. For example, in
Chapter 6, for the
JButton calculateB
, the following statement registers
the object
cbHandler
to listen for and register the action event:
calculateB.addActionListener(cbHandler);
Just as you create objects of the class that extends the
interface
ActionListener
to
handle action events, to handle window events you first create a class that implements the
interface
WindowListener
and then create and register objects of that class. You take
similar steps to handle mouse events.
In Chapter 6, to terminate the program when the close window button is clicked, we
used the method
setDefaultCloseOperation
with the predefined named constant
EXIT_ON_CLOSE
. If you want to provide your own code to terminate the program when
the window is closed, or if you want the program to take a different action when the
window closes, then you use the
interface
WindowListener
. That is, first you create
a class that implements the
interface
WindowListener
, provide the appropriate
definition of the method
windowClosed
, create an appropriate object of that class, and
then register the object created with the program.
Let's look at the definition of the
interface
WindowListener
:
public interface
WindowListener
{
1
1
void
windowActivated(WindowEvent e);
//This method executes when a window is activated.
void
windowClosed(WindowEvent e);
//This method executes when a window is closed.
void
windowClosing(WindowEvent e);
//This method executes when a window is closing,
//just before a window is closed.
void
windowDeactivated(WindowEvent e);
//This method executes when a window is deactivated.
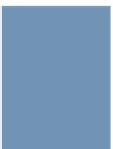
Search WWH ::

Custom Search