Java Reference
In-Depth Information
The following is an example of a method that returns a boolean value.
EXAMPLE 7-3 ROLLING A PAIR OF DICE
In this example, we write a method that rolls a pair of dice until the sum of the numbers
rolled is a specific number. We also want to know the number of times the dice are rolled
to get the desired sum.
The smallest number on each die is 1 and the largest number is 6. So the smallest sum of
the numbers rolled is 2 and the largest sum of the numbers rolled is 12. Suppose that we
have the following declarations:
int
die1;
int
die2;
int
sum;
int
rollCount = 0;
We use the random number generator, discussed in Chapter 5, to randomly generate a number
between 1 and 6. Then the following statement randomly generates a number between 1 and 6
and stores that number into
die1
, which becomes the number rolled by
die1
.
die1 = (
int
) (Math.random() * 6) + 1;
Similarly, the following statement randomly generates a number between 1 and 6 and
stores that number into
die2
, which becomes the number rolled by
die2
.
die2 = (
int
) (Math.random() * 6) + 1;
The sum of the numbers rolled by two dice is:
sum = die1 + die2;
Next, we determine whether
sum
contains the desired sum of the numbers rolled by the
dice. If
sum
does not contain the desired sum, then we roll the dice again. This can be
accomplished by the following
do
...
while
loop. (Assume that the
int
variable
num
contains the desired sum to be rolled.)
do
{
7
die1 = (
int
) (Math.random() * 6) + 1;
die2 = (
int
) (Math.random() * 6) + 1;
sum = die1 + die2;
rollCount++;
}
while
(sum != num);
We can now write the method
rollDice
that takes as a parameter the desired sum of the
numbers to be rolled and returns the number of times the dice are rolled to roll the desired sum.
public static int
rollDice(
int
num)
{
int
die1;
int
die2;
int
sum;
int
rollCount = 0;
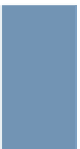

Search WWH ::

Custom Search